A lot of what programs do is transforming inputs into outputs. Take, for example, a piece of JavaScript code like this:
itemsToMarkup( items, viewType, galleryType ) {
let markup;
switch ( viewType ) {
case 'gallery':
if ( 'individual' === galleryType ) ) {
markup = getHTML( items );
} else {
markup = getShortcode( items );
}
break;
case 'image':
default:
markup = getHTML( items );
}
return markup;
}
What’s the purpose of this code? It takes some input data structures and outputs a markup, either proper HTML or a code to be processed by later stages of the pipeline. At the core, what we are doing is taking a decision based on the input’s state so it can be modeled as a decision tree:
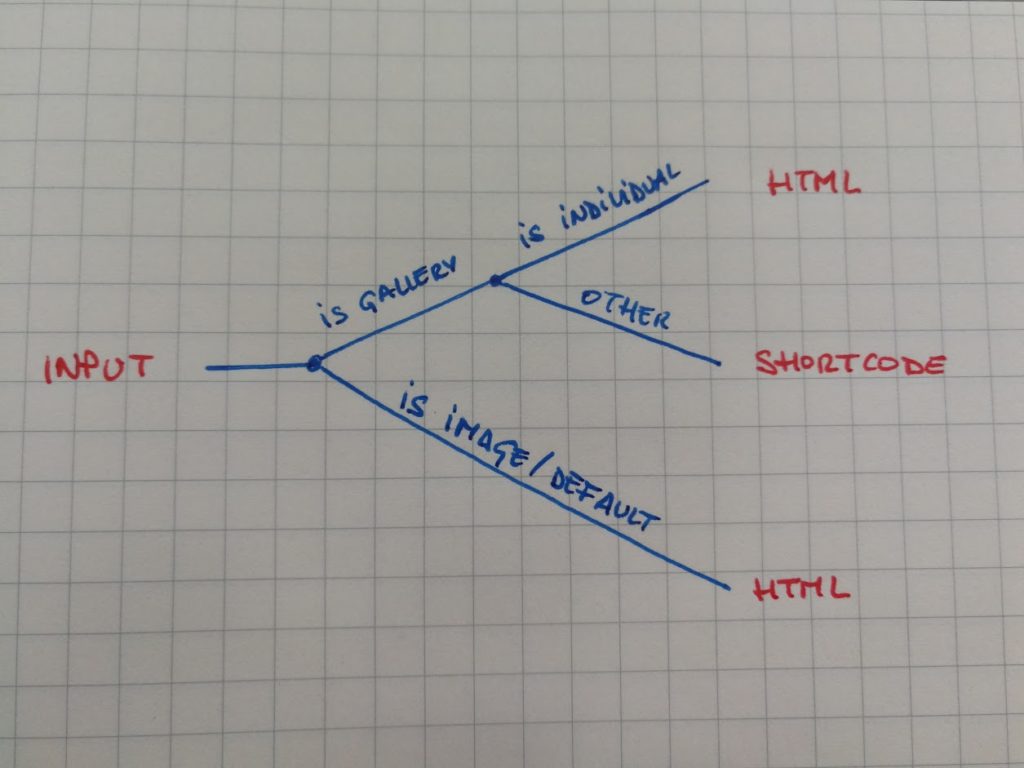
By restating the problem in a more simple language, the structure is made more evident. We are free of the biases that code as a language for thinking introduces (code size, good-looking indenting, a certain preference to use switch
or if
statements, etc). In this case, conflating the two checks into one reduces the tree depth and the number of leaves:
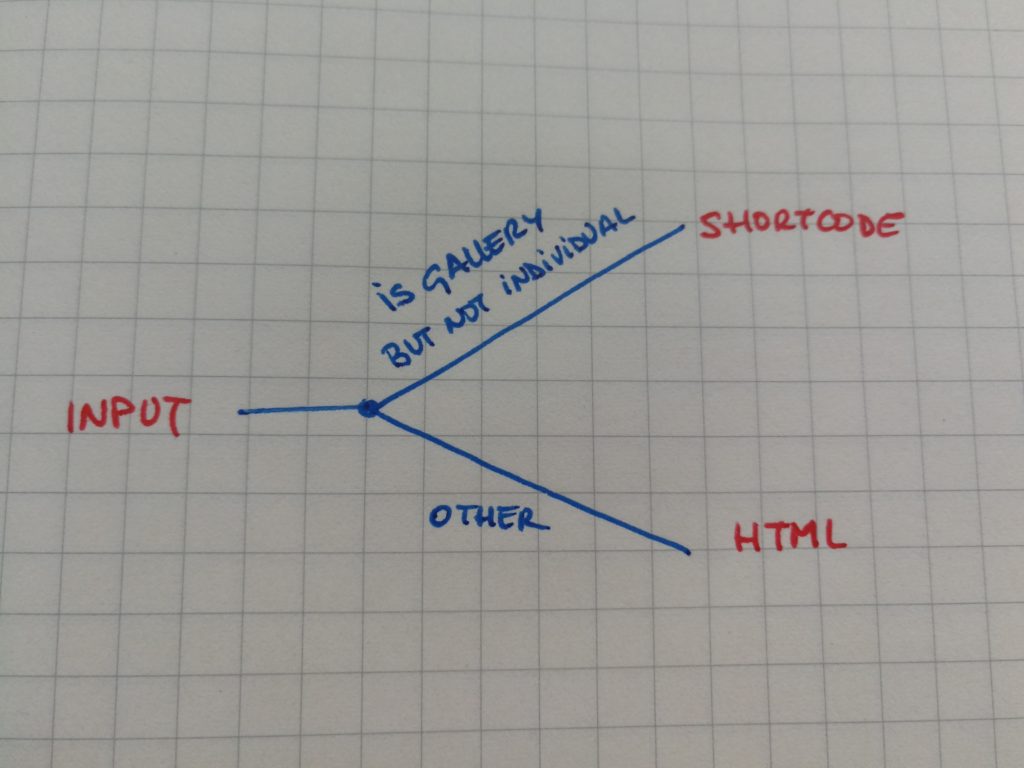
Which back to code could be something like:
itemsToMarkup( items, viewType, galleryType ) {
let markup;
const isGalleryButNotIndividual = ( view, gallery ) =>
view === 'gallery' &&
gallery !== 'individual';
if( isGalleryButNotIndividual( viewType, galleryType ) ) {
markup = getShortcode( items );
} else {
markup = getHTML( items );
}
return markup;
}
By having a simpler decision tree, the second piece of code makes the input/output mapping more explicit and concise.
Leave a Reply